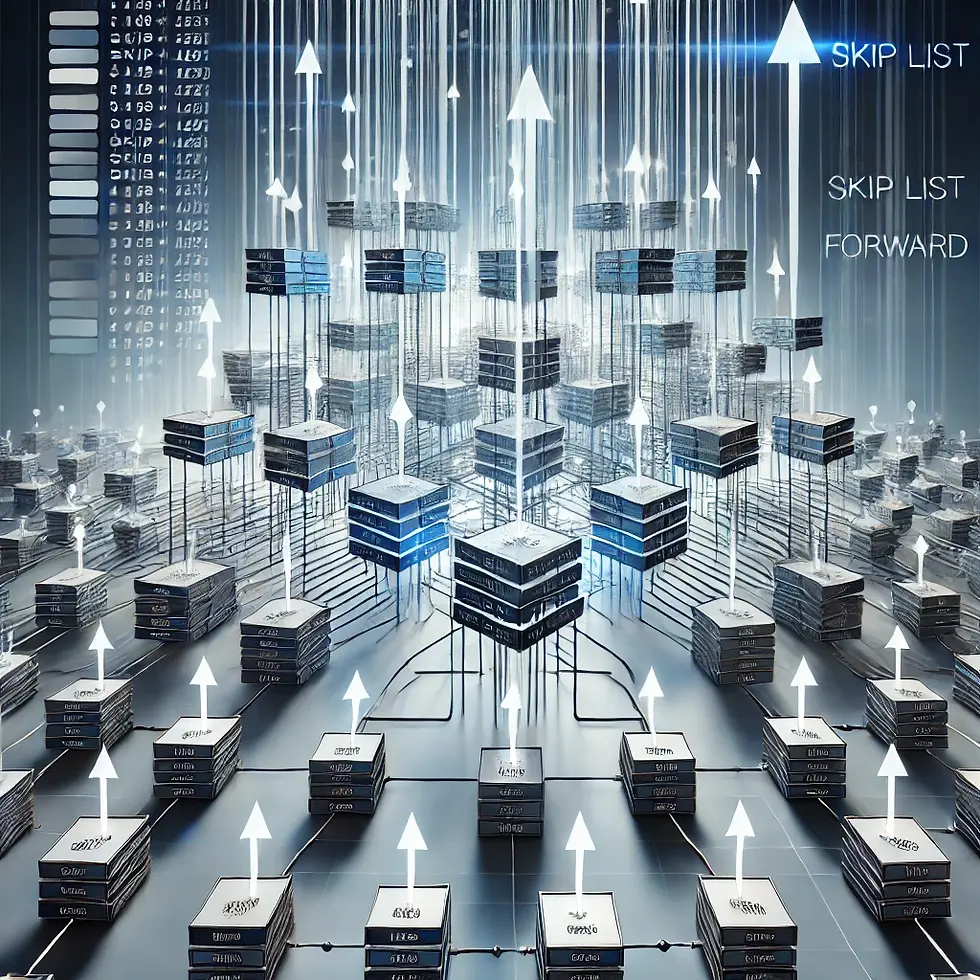
When it comes to efficient data structures for ordered lists, binary search trees (BSTs) and hash tables often steal the spotlight. But did you know there's a lightweight, probabilistic alternative that can compete with balanced trees like AVL or Red-Black Trees?
Enter the Skip List—a data structure that's as elegant as it is practical.
In this blog post, we’ll dive into the world of skip lists using a Python implementation. You’ll learn how skip lists work, why they’re efficient, and how to implement common operations like insertion, deletion, and lookup.
What is a Skip List?
A skip list is a probabilistic data structure that maintains elements in a sorted order, facilitating efficient search, insertion, and deletion operations. The unique aspect of a skip list lies in its use of multiple levels of linked lists, which enhances its performance compared to traditional data structures like binary trees. In a skip list, each level serves as an express lane that allows for "skipping" over several elements in the list below, thereby reducing the number of comparisons needed to locate an element. The foundational concept behind a skip list is that it combines the simplicity of linked lists with the efficiency of balanced trees. Each element in the skip list can appear in multiple levels, with the highest level containing the fewest elements. This hierarchical structure enables a logarithmic average time complexity for search operations, which is similar to that of balanced binary search trees. The probabilistic nature of the skip list comes into play during the insertion of new elements: when a new element is added, it is randomly assigned to a certain number of levels.
This randomness helps ensure that the levels remain balanced over time, which is crucial for maintaining the skip list's efficiency. The skip list's ability to maintain sorted order while allowing for fast operations is particularly advantageous in applications where dynamic data is prevalent.
Key Operations:
Insertion: Adds elements while maintaining sorted order.
Lookup: Searches for elements with logarithmic time complexity.
Deletion: Removes elements efficiently.
Why Use a Skip List?
Simplicity: Easier to implement than balanced trees.
Performance: Similar time complexity (O(log n)) for search, insert, and delete.
Space Efficiency: Flexible in memory usage.
Anatomy of a Skip List
Think of a skip list as a hierarchy of linked lists:
The bottom level contains all elements in sorted order.
Each higher level skips over more elements, reducing the number of nodes to traverse during a search.
A randomization process determines how many levels each element appears in, creating the probabilistic balance.
The Python Implementation
Here’s a full implementation of a skip list in Python. It includes the basic building blocks and operations.
The Node Class
Each node stores a value and forward pointers to nodes at higher levels:
class Node:
def __init__(self, value, level):
self.value = value
self.forward = [None] * (level + 1)
The SkipList Class
The skip list uses a random level generator to decide how many levels each node spans:
class SkipList:
def __init__(self, max_level):
self.max_level = max_level
self.header = Node(-1, max_level)
def random_level(self):
level = 0
while random.random() < 0.5 and level < self.max_level:
level += 1
return level
Core Operations
1. Insertion
Inserting a value involves:
Locating the correct position at each level.
Creating a new node.
Adjusting forward pointers.
def insert(self, value):
update = [None] * (self.max_level + 1)
current = self.header
for i in range(self.max_level, -1, -1):
while current.forward[i] and current.forward[i].value < value:
current = current.forward[i]
update[i] = current
random_level = self.random_level()
new_node = Node(value, random_level)
for i in range(random_level + 1):
new_node.forward[i] = update[i].forward[i]
update[i].forward[i] = new_node
2. Lookup
Search efficiently by skipping levels:
def lookup(self, value):
current = self.header
for i in range(self.max_level, -1, -1):
while current.forward[i] and current.forward[i].value < value:
current = current.forward[i]
if current.forward[i] and current.forward[i].value == value:
return True
return False
3. Deletion
Remove a value by updating pointers:
def delete(self, value):
update = [None] * (self.max_level + 1)
current = self.header
for i in range(self.max_level, -1, -1):
while current.forward[i] and current.forward[i].value < value:
current = current.forward[i]
update[i] = current
current = current.forward[0]
if current and current.value == value:
for i in range(len(current.forward)):
if update[i].forward[i] != current:
break
update[i].forward[i] = current.forward[i]
Visualizing the Skip List
The display() method prints the structure level by level:
def display(self):
for i in range(self.max_level + 1):
print(f"Level {i}: ", end="")
node = self.header.forward[i]
while node:
print(node.value, end=" ")
node = node.forward[i]
print()
Running the Skip List
Here’s a simple interactive demo to test the skip list:
def main():
skip_list = SkipList(max_level=5)
while True:
operation = input("Enter operation (insert, delete, lookup) and value, or 'exit' to stop: ").split()
if operation[0] == 'exit':
break
op, val = operation[0], int(operation[1])
if op == 'insert':
skip_list.insert(val)
elif op == 'delete':
skip_list.delete(val)
elif op == 'lookup':
skip_list.lookup(val)
skip_list.display()
Why Skip Lists Matter
Skip lists are a fascinating data structure that offer significant advantages in specific computational scenarios, particularly when the complexities of maintaining balanced trees become overwhelming or when the inherent unordered nature of hash tables proves to be a limitation. This duality of functionality makes skip lists an appealing choice for a variety of applications, especially in modern computing environments. Their unique design allows for efficient searching, insertion, and deletion operations, all while maintaining a sorted order of elements, which is crucial for many applications.
In the realm of databases, skip lists are often employed as an alternative to traditional B-trees or other balanced tree structures. The reason for this preference lies in their simpler implementation and the reduced overhead associated with maintaining balance. When dealing with large datasets, the performance benefits of skip lists can be particularly pronounced, as they allow for logarithmic time complexity for search operations, akin to that of balanced trees, but with less stringent requirements for restructuring the data on updates.
Final Thoughts
Skip lists offer a perfect blend of simplicity and efficiency. Whether you’re designing a distributed database or experimenting with algorithms, understanding skip lists is a must-have tool in your data structures arsenal. Try the implementation above and see how it works for yourself!
Comentarios