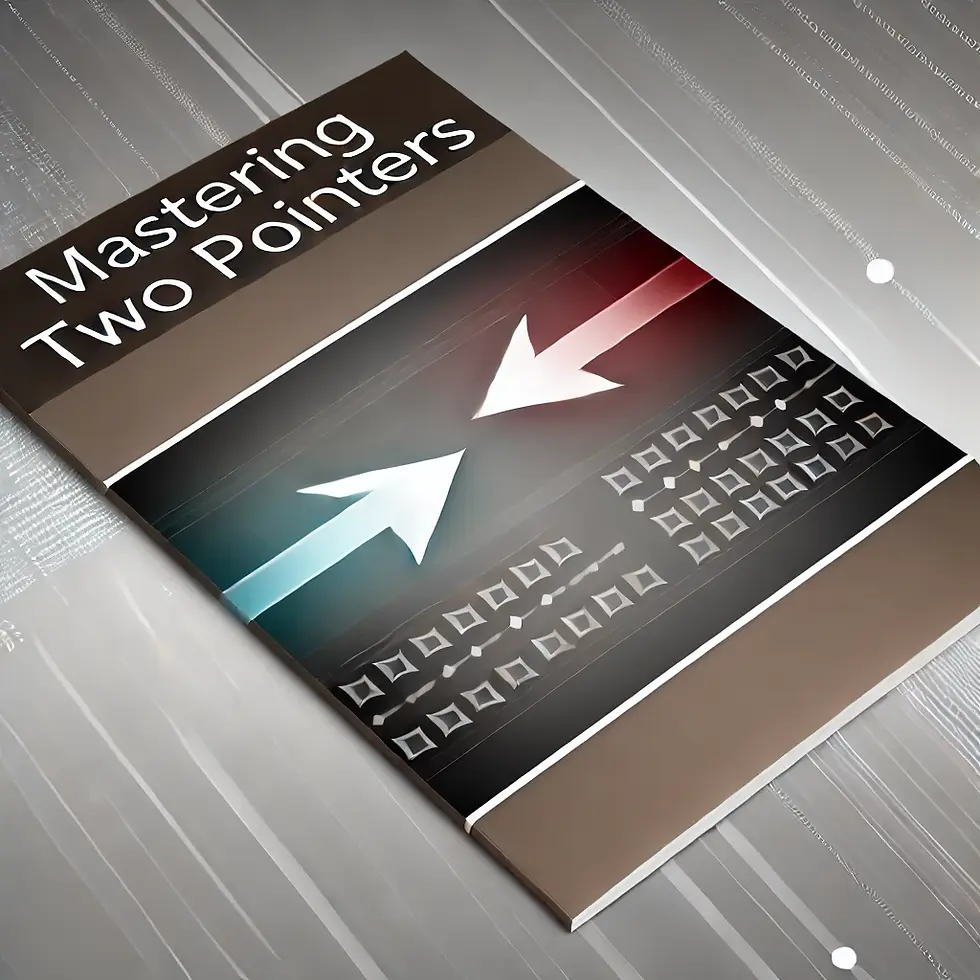
The two-pointer technique is a powerful algorithmic strategy used to solve problems on arrays or strings with O(n) time complexity. It involves using two pointers that traverse the data structure in a specific way to achieve optimal performance. This guide will walk you through the basics, examples, and how to master this approach.
What is the Two-Pointer Technique?
The two-pointer technique uses two indices (or "pointers") to process input data. These pointers can move:
In the same direction, often to calculate ranges or manage sliding windows.
In opposite directions, typically for searching or comparing elements.
When Should You Use Two Pointers?
Two pointers are useful when:
You need to optimize problems involving subarrays, substrings, or pairs.
The problem has sorted arrays, where opposite-direction pointers are common.
You need to efficiently search for combinations (e.g., pairs, triplets).
Types of Two-Pointer Problems
Sliding Window Problems (Same Direction):
Used to find substrings or subarrays with specific properties.
Example: Finding the longest substring without repeating characters.
Opposite Direction Problems:
Typically used to find pairs of elements (e.g., summing to a target).
Example: Checking if a string is a palindrome.
Example 1: Longest Substring Without Repeating Characters
Problem:
Find the length of the longest substring in a string s that contains no repeating characters.
Approach:
Use a sliding window with two pointers:
Start both pointers at the beginning of the string.
Move the right pointer to expand the window and add characters to a "seen" set.
If a duplicate character is encountered, move the left pointer to shrink the window.
Track the maximum window size.
Code:
def length_of_longest_substring(s):
seen = set()
left = 0
max_length = 0
for right in range(len(s)):
while s[right] in seen: # Remove duplicates
seen.remove(s[left])
left += 1
seen.add(s[right])
max_length = max(max_length, right - left + 1)
return max_length
Example 2: Two Sum in a Sorted Array
Problem:
Given a sorted array, find two numbers that add up to a target.
Approach:
Use two pointers:
Start one pointer at the beginning (left) and the other at the end (right).
Calculate the sum of the elements at the two pointers.
If the sum equals the target, return the indices.
If the sum is less than the target, move the left pointer rightward.
If the sum is greater, move the right pointer leftward.
Code:
def two_sum(nums, target):
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target:
return [left, right]
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
Example 3: Remove Element
Problem:
Remove all occurrences of a value val in an array in-place and return the new length of the array.
Approach:
Use one pointer (k) to keep track of the position for non-val elements.
Iterate through the array:
If the current element is not val, place it at position k and increment k.
Code:
def remove_element(nums, val):
k = 0
for i in range(len(nums)):
if nums[i] != val:
nums[k] = nums[i]
k += 1
return k
Tips for Mastering Two Pointers
Visualize the Process: Draw examples to understand how the pointers move and interact with the data.
Identify Patterns: Common problems like sliding windows and pair matching are great starting points.
Practice Edge Cases: Test your solutions with edge cases like empty arrays or strings.
Understand Constraints: Use the problem's constraints to optimize your solution (e.g., sorted input).
Why Two Pointers?
Two pointers reduce the time complexity by avoiding nested loops. Instead of checking all combinations, they allow you to iterate through the data structure efficiently.
Code Templates for Two-Pointer Problems
Here are some commonly used templates for solving two-pointer problems. These templates provide a quick starting point for various scenarios, such as sliding windows, finding pairs, and more.
1. Sliding Window (Same Direction)
Use Case:
Find the length of a substring or subarray with specific properties, such as distinct characters or a maximum sum.
def sliding_window(nums, condition):
left = 0
result = 0
for right in range(len(nums)):
# Update the window with nums[right]
# Example: Add nums[right] to a running sum or set
while not condition: # If the window violates a condition, shrink it
# Remove nums[left] from the window
left += 1
# Update the result
result = max(result, right - left + 1)
return result
2. Opposite Direction (Sorted Input)
Use Case:
Find pairs or triplets that satisfy specific conditions (e.g., two-sum, check for palindrome).
def two_pointers_opposite(nums, target):
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target: # Found the condition
return [left, right]
elif current_sum < target:
left += 1 # Move left pointer to increase the sum
else:
right -= 1 # Move right pointer to decrease the sum
return [] # No solution found
3. Two Pointers for Removing/Filtering Elements
Use Case:
Remove duplicates, filter elements in-place, or rearrange an array.
def remove_element(nums, val):
k = 0 # Pointer for the next position of non-val elements
for i in range(len(nums)):
if nums[i] != val:
nums[k] = nums[i] # Place non-val element at index k
k += 1
return k # k is the count of non-val elements
4. Finding the Longest Substring Without Repeating Characters
Use Case:
Find the length of the longest substring that meets specific criteria (e.g., no repeating characters).
def longest_substring(s):
seen = set()
left = 0
max_length = 0
for right in range(len(s)):
while s[right] in seen: # If character repeats, shrink the window
seen.remove(s[left])
left += 1
seen.add(s[right])
max_length = max(max_length, right - left + 1)
return max_length
5. Merging Two Sorted Arrays
Use Case:
Merge two sorted arrays in sorted order using two pointers.
def merge_sorted_arrays(nums1, nums2):
i, j = 0, 0
result = []
while i < len(nums1) and j < len(nums2):
if nums1[i] < nums2[j]:
result.append(nums1[i])
i += 1
else:
result.append(nums2[j])
j += 1
# Add remaining elements
result.extend(nums1[i:])
result.extend(nums2[j:])
return result
6. Partitioning Arrays
Use Case:
Rearrange an array based on conditions (e.g., move all zeros to the end).
def partition_array(nums, condition):
left = 0 # Pointer for placing elements that meet the condition
for right in range(len(nums)):
if condition(nums[right]): # If nums[right] satisfies the condition
nums[left], nums[right] = nums[right], nums[left]
left += 1
return nums # Partitioned array
7. Two-Sum Closest (Variant of Two-Sum)
Use Case:
Find two numbers whose sum is closest to a given target.
def two_sum_closest(nums, target):
nums.sort() # Ensure the array is sorted
left, right = 0, len(nums) - 1
closest_sum = float('inf')
while left < right:
current_sum = nums[left] + nums[right]
if abs(target - current_sum) < abs(target - closest_sum):
closest_sum = current_sum
if current_sum < target:
left += 1
else:
right -= 1
return closest_sum
Tips for Using Templates
Understand the Condition: Before applying a template, make sure you know the condition your problem is solving (e.g., sum constraints, substring uniqueness).
Optimize with Early Exits: In problems like finding pairs or substrings, you can often return early once the desired condition is met.
Adjust Pointers Carefully: Ensure that pointers move correctly based on the condition being checked (e.g., incrementing or decrementing).
These templates can be adapted to solve many common two-pointer problems efficiently. Practice applying them to various scenarios to build a strong understanding!
Conclusion
The two-pointer technique is an essential tool for solving many algorithmic problems. By practicing problems like sliding windows and pair searches, you'll gain confidence in applying this approach to various challenges.
Remember, mastering two pointers is all about practice and recognizing the right situations to use it. Start small, experiment with different problems, and soon, you'll be solving complex challenges with ease!
Comments